import numpy as np
import matplotlib.pyplot as plt
Práctica 2: Optimizar función¶
Usaremos el paquete Pygad para hallar óptimos de una función diferenciable.
Objetivo¶
Hallar el óptimo de la función
\[
f(x,y)=(x-2)^2+(y-2)^2
\]
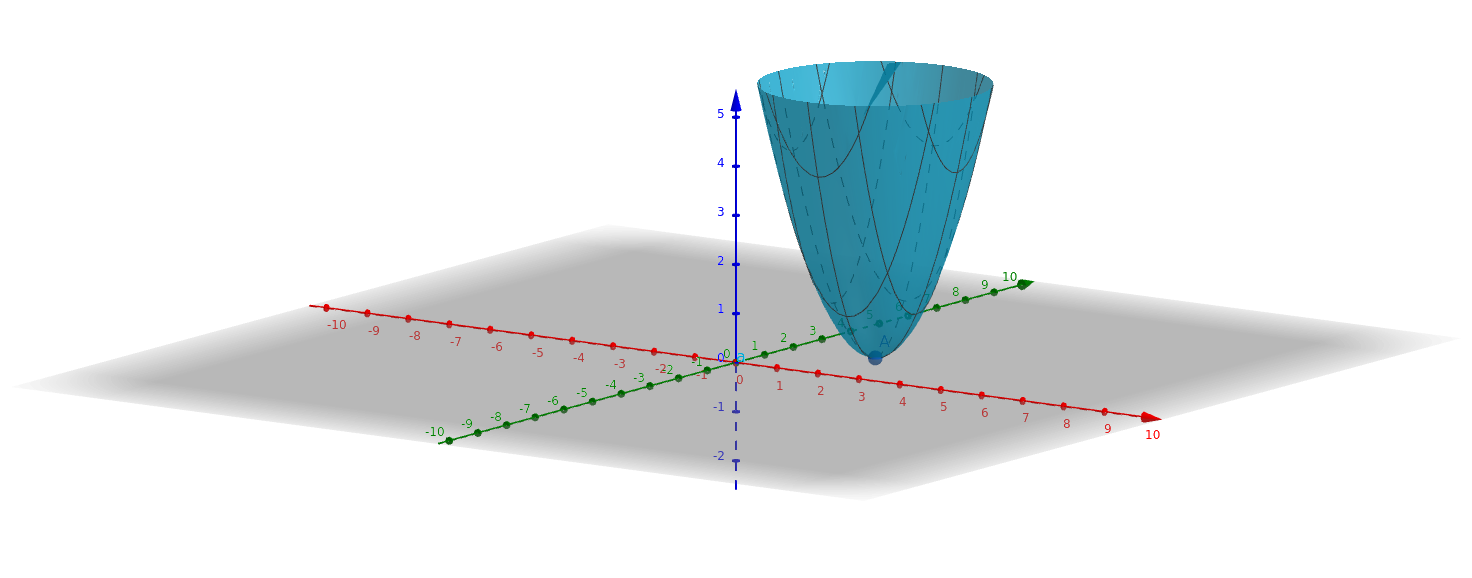
Fig. 46 Función de dos variables¶
Sabemos que \(f\) tiene un mínimo (local) en el punto \((2,2)\). ¿Cómo?
Vamos a comprobar si el algoritmo genético es capaz de hallar dicho punto.
def fitness_func(solution, solution_idx):
x,y=solution
fvalue = (x-2)**2+(y-2)**2
# maximization problem
return 1/fvalue #attention possible inf
fitness_function = fitness_func
#https://pygad.readthedocs.io/en/latest/README_pygad_ReadTheDocs.html#life-cycle-of-pygad
def on_generation(ga):
print('Generación',ga.generations_completed)
print(ga.population)
def on_start(ga):
print('Starting generation',ga.generations_completed)
print(ga.population)
Parámetros¶
https://pygad.readthedocs.io/en/latest/README_pygad_ReadTheDocs.html#pygad-ga-class
num_generations = 25
# num_parents_mating: Number of solutions to be selected as parents.
num_parents_mating = 4
# sol_per_pop: Number of solutions (i.e. chromosomes) within the population.
#This parameter has no action if initial_population parameter exists.
sol_per_pop = 20
population_list=[[10,10],
[-10,-10],
[10,-10],
[-10,10]
]
#num_genes: Number of genes in the solution/chromosome.
#This parameter is not needed if the user feeds the initial population to the initial_population parameter
num_genes = 2
# mutation_num_genes=None: Number of genes to mutate which defaults to None meaning that no number is specified.
mutation_num_genes = 2
#init_range_low=-4: The lower value of the random range from which the gene values
#in the initial population are selected.
#init_range_low defaults to -4.
init_range_low=-10
init_range_high=10
#https://pygad.readthedocs.io/en/latest/README_pygad_ReadTheDocs.html#supported-parent-selection-operations
parent_selection_type= "sss"
#keep_parents=-1: Number of parents to keep in the current population.
#-1 (default) means to keep all parents in the next population.
#0 means keep no parents in the next population.
#A value greater than 0 means keeps the specified number of parents in the next population.
#Note that the value assigned to keep_parents cannot be < - 1
#or greater than the number of solutions within the population sol_per_pop.
keep_parents = 1
#https://pygad.readthedocs.io/en/latest/README_pygad_ReadTheDocs.html#data-type-for-all-genes-without-precision
gene_type=float
#https://pygad.readthedocs.io/en/latest/README_pygad_ReadTheDocs.html#supported-crossover-operations
crossover_type = "two_points"
#https://pygad.readthedocs.io/en/latest/README_pygad_ReadTheDocs.html#supported-mutation-operations
mutation_type="random"
# mutation_percent_genes="default": Percentage of genes to mutate.
#It defaults to the string "default" which is later translated into the integer 10
#which means 10% of the genes will be mutated.
#It must be >0 and <=100.
#Out of this percentage, the number of genes to mutate is deduced
#which is assigned to the mutation_num_genes parameter.
mutation_percent_genes = 10
Definición del algoritmo genético¶
import pygad
ga_instance = pygad.GA(num_generations=num_generations,
sol_per_pop=sol_per_pop,
num_parents_mating=num_parents_mating,
fitness_func=fitness_function,
num_genes=num_genes,
mutation_num_genes=mutation_num_genes,
init_range_low=init_range_low,
init_range_high=init_range_high,
parent_selection_type=parent_selection_type,
gene_type=gene_type,
keep_parents=keep_parents,
crossover_type=crossover_type,
mutation_type=mutation_type,
mutation_percent_genes=mutation_percent_genes,
save_solutions=True,
on_generation=on_generation,
on_start=on_start)
/home/carlos/.local/lib/python3.8/site-packages/pygad/pygad.py:795: UserWarning: Use the 'save_solutions' parameter with caution as it may cause memory overflow when either the number of generations, number of genes, or number of solutions in population is large.
if not self.suppress_warnings: warnings.warn("Use the 'save_solutions' parameter with caution as it may cause memory overflow when either the number of generations, number of genes, or number of solutions in population is large.")
Llamada a la optimización¶
ga_instance.run()
Starting generation 0
[[ 7.77074797 -9.993278 ]
[ 0.77707472 -6.55508267]
[ 9.55178371 4.95585868]
[ 5.00139492 -8.06096971]
[-2.30736975 -4.41778046]
[-3.97559529 -8.4973897 ]
[ 7.84142503 7.46115622]
[ 9.47860386 6.92645059]
[ 2.26616064 4.76420286]
[ 1.08297713 -2.04498717]
[ 6.00683764 -8.73304441]
[-7.76945864 -6.21953725]
[ 2.50693975 6.40256302]
[ 7.58579474 5.32009488]
[-5.69170879 5.2875297 ]
[-1.24518912 -2.40853254]
[ 1.02575446 4.915821 ]
[-9.2370701 -7.80750271]
[ 8.03857272 -2.69995115]
[-8.17980224 -8.09865977]]
Generación 1
[[ 2.26616064 4.76420286]
[ 1.38120634 5.45532496]
[ 0.8776571 4.55578649]
[ 1.79250393 6.70864803]
[ 3.40078971 4.27187173]
[ 0.02972593 3.94791191]
[ 0.45328964 4.44048828]
[ 2.17426912 -2.88747213]
[ 1.87139621 6.41950206]
[ 1.54527642 4.87616058]
[ 1.07960658 -1.79076511]
[ 1.63752786 6.65562806]
[ 3.12162486 4.58784829]
[ 2.91255434 4.54377365]
[ 0.44645913 5.28108289]
[ 1.09827075 5.476014 ]
[ 2.25038978 6.16111446]
[ 0.94825839 5.6538104 ]
[ 0.34282672 -2.71619707]
[ 1.52943325 5.51556254]]
Generación 2
[[ 3.40078971 4.27187173]
[ 2.36023993 3.87374925]
[ 2.13725397 4.58813321]
[ 1.29860271 4.88409804]
[ 3.25160979 3.6605809 ]
[ 2.01597314 4.54288996]
[ 3.31338618 4.60200044]
[ 0.67688076 5.71279541]
[ 2.64596806 5.02254834]
[ 3.54753292 3.92288984]
[ 2.13537351 2.9751686 ]
[ 2.8079269 3.13666913]
[ 4.16436232 4.58878403]
[ 4.38036196 4.85899832]
[ 3.77762531 4.35563829]
[ 2.03753617 4.36471559]
[ 4.07475582 4.57361823]
[ 3.49472066 4.87022877]
[ 0.12952556 3.80031574]
[-0.51787423 5.29861426]]
Generación 3
[[2.13537351 2.9751686 ]
[2.54958308 2.17130443]
[1.85556065 4.65987119]
[2.60148151 4.50749666]
[3.07668878 3.14545876]
[1.99043571 2.81846035]
[3.59964282 4.30158506]
[2.23147806 3.37666822]
[1.79007076 4.12780223]
[2.42656566 2.66587243]
[3.76626087 2.98173253]
[3.14498153 3.10013756]
[3.09145169 4.29594931]
[1.83288545 2.34372507]
[2.41084076 4.1469601 ]
[3.34944178 3.18495309]
[4.05127157 3.16433663]
[3.40657197 3.67892578]
[3.40151897 4.10296652]
[2.60378966 3.00008203]]
Generación 4
[[1.83288545 2.34372507]
[2.21783267 2.76303454]
[2.23716967 2.00990345]
[2.21097018 2.79504256]
[2.9058182 2.91836925]
[1.82596487 1.22399516]
[2.9541403 3.11048536]
[2.565547 3.41200574]
[1.42573358 2.66679882]
[2.10805639 1.94343416]
[2.31941409 1.42959055]
[1.46379686 2.7887473 ]
[1.82558027 2.03327083]
[2.50783302 1.97128902]
[2.02712797 1.72714903]
[2.15637164 2.85847484]
[1.35861357 1.41624282]
[2.75289983 2.34109837]
[1.65221973 2.20315472]
[1.06431212 3.16895302]]
Generación 5
[[2.10805639 1.94343416]
[1.97646886 2.62284956]
[1.43266624 1.39915407]
[2.18348705 1.36581987]
[1.21826676 2.60276274]
[2.4066372 2.16433978]
[1.99467716 2.5797849 ]
[1.9228773 1.70065486]
[1.27654911 0.89781546]
[1.96027487 1.3808758 ]
[2.59442823 2.0478387 ]
[2.76860997 1.54003297]
[2.9816345 1.94616369]
[2.5568453 1.48164413]
[1.29935227 1.46044185]
[2.13581007 2.29248131]
[1.53920224 1.34356163]
[1.24347596 2.30084008]
[2.58378785 2.01281895]
[2.82900243 1.2485593 ]]
Generación 6
[[2.10805639 1.94343416]
[2.80708979 1.98560883]
[1.68099746 1.30534719]
[2.55550753 1.74042327]
[1.41254439 1.5617493 ]
[3.10046725 1.02891005]
[2.35699782 2.99419163]
[3.14857675 1.81925898]
[1.88659415 2.08007981]
[2.57055116 1.14453882]
[0.93135036 1.30250012]
[2.34496319 2.56796693]
[1.47737378 2.11329546]
[1.37976184 2.01405467]
[2.77405191 1.36760793]
[2.00104774 2.30160823]
[2.90393678 2.73084469]
[2.27039692 1.66310747]
[2.33638226 1.07203146]
[2.33078239 2.94155111]]
Generación 7
[[2.10805639 1.94343416]
[1.18417191 1.54040961]
[2.07128937 1.82962318]
[2.63677955 1.83281029]
[1.58459675 2.54131563]
[1.57461393 2.07315262]
[2.17855281 2.79971265]
[1.50531189 1.63834407]
[1.95756734 2.34197846]
[2.57445642 1.59476518]
[1.97231462 2.23087963]
[1.97326901 3.13810914]
[1.38029879 1.24084515]
[2.29761857 0.98941682]
[2.48463866 1.31556272]
[2.79441709 2.23958123]
[2.20301347 2.20671487]
[1.37012507 2.40944176]
[2.93659989 2.9162381 ]
[2.95498728 2.93022749]]
Generación 8
[[2.10805639 1.94343416]
[1.54138505 2.7450156 ]
[2.43845078 2.54634146]
[2.70289698 2.7897963 ]
[2.70322265 1.37394122]
[1.8299921 1.59792273]
[1.2141407 1.54348715]
[1.5779138 1.54211039]
[2.82631491 1.76872398]
[2.19877675 1.49158015]
[2.30644094 2.2753554 ]
[2.86601208 3.15617545]
[2.42685611 2.20480749]
[1.76513456 2.58478555]
[1.56207443 1.64397105]
[2.7197463 2.52611442]
[2.29611113 2.02345776]
[2.1069117 1.3110202 ]
[1.11486569 1.34552192]
[2.47526633 2.51928597]]
Generación 9
[[2.10805639 1.94343416]
[1.25192251 1.97027477]
[3.18979975 1.77626404]
[2.2157938 2.83519467]
[2.1618359 2.01769964]
[2.38105264 2.43365011]
[3.06542278 1.13962971]
[2.86749749 2.00446075]
[2.30571681 1.9723597 ]
[3.05873024 2.05932457]
[2.00725563 1.46619937]
[2.49893786 2.20577878]
[2.0910504 2.54309628]
[2.0578995 2.68104415]
[2.01359704 1.41007136]
[1.55744741 1.34390151]
[1.43581298 1.09182003]
[1.59082978 1.66166922]
[2.35757412 2.04940626]
[2.48445497 1.14149893]]
Generación 10
[[2.10805639 1.94343416]
[2.42416765 2.71221095]
[1.69593721 2.15275118]
[2.49056576 1.50931414]
[2.50727984 2.63014399]
[1.99603299 1.6804319 ]
[1.8261797 2.59489358]
[2.7260299 2.62021525]
[2.92172439 1.42979341]
[2.70980428 1.15288538]
[3.10074273 2.12671208]
[1.40669496 1.67802996]
[3.13162205 1.67173915]
[1.65437239 1.01828821]
[1.40136027 2.05797895]
[2.80083734 2.09594436]
[3.06119556 2.34855441]
[1.42481174 2.25279327]
[1.44332482 1.05728443]
[1.64323968 2.10759596]]
Generación 11
[[2.10805639 1.94343416]
[1.89582544 1.28414766]
[1.4840438 2.04401967]
[1.50598101 2.45218042]
[2.71193296 2.38436675]
[2.92824992 1.8308885 ]
[0.94904052 2.56631653]
[2.01906762 2.00136723]
[2.45497746 1.43249517]
[1.82722614 1.57675833]
[1.53885646 1.08214912]
[2.11703609 1.57625947]
[2.52860043 1.60922235]
[1.77122788 2.01320367]
[1.92222479 2.86708726]
[1.1267151 1.94379939]
[1.57731184 1.58571071]
[2.33540005 1.85698287]
[1.47124463 1.82027682]
[1.56393869 2.3522804 ]]
Generación 12
[[2.01906762 2.00136723]
[2.5465634 2.46252529]
[1.14904949 2.46070863]
[1.80749047 2.45621952]
[1.18908349 1.68400026]
[1.36508567 2.8786733 ]
[1.63778211 2.42696666]
[0.98041819 1.51938916]
[2.49456637 1.05979229]
[1.70988247 1.84648911]
[1.17005656 1.29041478]
[2.34871211 1.96747645]
[1.4484654 1.9605542 ]
[1.98444865 1.82985871]
[2.06880884 1.48134357]
[1.97783512 2.13311332]
[2.83526181 1.61750364]
[2.59131243 2.15694138]
[2.78826408 2.00537359]
[2.22910328 1.13686249]]
Generación 13
[[2.01906762 2.00136723]
[2.68123444 1.16442305]
[2.24643653 2.05267588]
[0.99723785 2.38311939]
[2.24794704 1.02746882]
[2.56297849 1.51453417]
[1.42776331 2.03093422]
[1.80227419 2.47794124]
[1.33484153 1.87691064]
[2.03637888 1.00678925]
[1.14901744 1.70084188]
[2.09944203 1.12924132]
[2.40073495 1.51053021]
[2.58261602 1.96940553]
[1.65708642 2.18778542]
[1.29762394 1.82010771]
[0.86910783 1.86246135]
[2.02428859 2.25281352]
[1.43689633 2.25764259]
[1.13531842 1.66539157]]
Generación 14
[[2.01906762 2.00136723]
[1.18024791 2.85641351]
[1.14938479 2.12781167]
[2.20449929 1.34043316]
[2.01445916 2.72237135]
[2.49307048 1.97132033]
[1.24902115 1.64754329]
[1.89003782 2.79267717]
[1.65342181 2.75823949]
[3.13003703 2.1673361 ]
[1.59048968 3.02849224]
[1.72245239 2.93163056]
[2.22359953 1.53409875]
[1.67450161 2.61622251]
[3.22666499 3.10298211]
[2.79589993 1.4690792 ]
[1.05773949 1.05426973]
[1.82233821 2.34755788]
[1.7458177 1.73683328]
[1.68670834 1.23961201]]
Generación 15
[[2.01906762 2.00136723]
[2.95339416 1.01349462]
[2.61237666 1.08138922]
[1.87279732 1.81170667]
[2.08504222 2.23017013]
[1.3262427 1.83763438]
[2.63260172 2.9017405 ]
[2.32039198 2.38105418]
[2.50935477 2.12757086]
[2.21220429 2.06378226]
[1.62978673 1.21297061]
[1.79260719 1.74936475]
[1.49560859 1.95581847]
[1.92590135 1.74090574]
[2.11449455 2.540842 ]
[2.8410155 2.72317549]
[1.5331672 2.15764147]
[1.58956247 1.09150497]
[2.55874567 1.42590411]
[0.87723786 1.2042689 ]]
Generación 16
[[2.01906762 2.00136723]
[1.45133949 2.69240966]
[2.59074847 2.79464597]
[2.38381501 1.02347633]
[2.45741889 2.93371594]
[1.32919128 2.1029347 ]
[1.75925571 2.05666773]
[1.72913147 1.79408088]
[1.04285226 3.19452496]
[1.02696839 2.2792156 ]
[1.91405278 1.10410046]
[1.62143411 2.09598754]
[1.1268565 2.91488823]
[1.49305252 1.78394339]
[1.45118954 2.37347948]
[2.45588107 2.25816832]
[2.13241266 1.43201726]
[2.50450806 2.4843678 ]
[1.61181574 2.48936123]
[1.17895379 1.27465777]]
Generación 17
[[2.01906762 2.00136723]
[2.59557603 2.30712837]
[2.22295313 1.4878863 ]
[2.04328934 2.78927886]
[1.17902267 1.65384854]
[1.50742329 1.05793881]
[1.25813158 2.03810946]
[2.50765771 1.29664223]
[1.46685539 2.45822522]
[1.93814687 1.17736342]
[1.68701843 1.34831068]
[1.83432197 2.37705001]
[2.33476836 1.54606555]
[2.15869234 2.14873867]
[1.3839093 2.12366771]
[1.60964041 2.71100873]
[2.01450181 1.61234875]
[0.99492093 1.67311184]
[2.21929416 3.02672461]
[2.11378352 2.59605105]]
Generación 18
[[2.01906762 2.00136723]
[2.14691958 1.80303653]
[2.31091478 1.25614426]
[2.15166723 2.30832266]
[2.37052263 2.91975064]
[1.85713487 1.45163354]
[1.08540888 2.84935768]
[2.54194346 2.37166102]
[1.1276412 2.95633671]
[1.52919572 1.80022691]
[2.88432788 1.98507103]
[0.8410048 1.98742941]
[1.08323586 2.32991281]
[2.29669855 2.92041372]
[2.87770567 1.35362997]
[2.24682755 1.42894372]
[0.95643031 1.7614021 ]
[2.78026356 1.19237671]
[2.06882243 1.33006091]
[1.84882979 1.75911709]]
Generación 19
[[2.01906762 2.00136723]
[1.61557324 1.44450548]
[2.95473167 1.62279622]
[2.41034755 2.3403198 ]
[1.83881659 2.19973018]
[1.15867396 2.13817423]
[2.25212273 2.14539261]
[1.50592259 2.63000506]
[2.72187063 2.07657565]
[2.81493387 2.84436027]
[1.23201168 1.06350204]
[2.02205381 1.65406518]
[1.61156082 2.46729695]
[1.31993181 2.23478215]
[2.20185797 1.8209328 ]
[1.34731281 2.29464993]
[2.66936653 3.22664561]
[1.69006559 2.52390304]
[0.8493063 1.65331023]
[1.15727785 2.76625669]]
Generación 20
[[2.01906762 2.00136723]
[1.56832341 1.99218994]
[1.48785465 2.59556384]
[3.0535674 1.44353567]
[1.51509973 1.79656055]
[2.42311473 2.78238375]
[2.49663166 2.55400264]
[1.40375342 2.97070424]
[1.5366427 1.58392409]
[2.59936595 2.9841612 ]
[1.74984031 1.42449496]
[3.11527264 2.21177325]
[2.07492398 2.19485117]
[2.30750428 1.80065418]
[1.07381949 2.0671856 ]
[1.43345873 1.2295519 ]
[2.10656323 2.05689202]
[2.47840869 1.30204642]
[1.53885975 1.30080661]
[2.22664546 2.68832918]]
Generación 21
[[2.01906762 2.00136723]
[1.80815189 2.17004013]
[1.23038487 1.30851951]
[1.77348815 2.81814826]
[1.39151498 2.35371943]
[1.83832755 2.44755916]
[2.38523995 1.96897374]
[1.48734171 3.04761984]
[1.98702724 1.38966759]
[2.18308304 1.39739143]
[1.86821411 1.9020075 ]
[2.63831727 1.40001872]
[1.88847141 1.75482232]
[1.65245937 2.5565612 ]
[1.89181591 2.95003851]
[2.81601078 2.3305546 ]
[1.88512243 1.53924542]
[1.3391833 1.26804356]
[1.41302504 2.10566022]
[3.1602463 2.04636427]]
Generación 22
[[2.01906762 2.00136723]
[1.65459131 1.52959988]
[2.0111805 1.13430144]
[1.48320491 1.21075441]
[2.44449116 1.59166142]
[2.26967961 1.73792043]
[1.03057098 3.05436402]
[2.38309007 0.79327191]
[1.60458104 2.63113709]
[2.66249661 1.10566946]
[2.46174031 1.84423644]
[1.76174978 1.19928374]
[1.03785338 1.50491035]
[1.22405966 1.86694438]
[2.56926202 2.31311656]
[2.86485038 2.80082988]
[2.19699796 2.6194564 ]
[1.13433535 2.23736639]
[2.37096172 3.03341879]
[1.47873551 2.66023511]]
Generación 23
[[2.01906762 2.00136723]
[1.0527408 0.95474073]
[2.22459759 1.66133359]
[0.9653862 2.29232106]
[2.84623209 0.61302715]
[2.38986482 1.25489925]
[1.30389092 1.457198 ]
[1.94637787 2.50809781]
[1.78864009 0.99924698]
[1.99393244 2.46213114]
[1.75235119 2.58505346]
[2.31705726 2.77922237]
[2.45008948 1.69651348]
[1.59411208 2.0202542 ]
[2.75442797 2.4006681 ]
[1.37979627 1.78893922]
[1.44024977 1.10776459]
[1.17318205 0.91505676]
[2.43748847 0.772807 ]
[0.83359615 1.76754466]]
Generación 24
[[2.01906762 2.00136723]
[2.68486518 2.85151842]
[2.30901868 1.55611547]
[2.18937785 1.05457244]
[1.33246288 2.18893772]
[1.26292457 1.90732084]
[1.38268784 1.76466356]
[1.84966969 1.8449808 ]
[2.81345231 1.72478865]
[2.23129881 2.60173029]
[2.40272782 1.32430806]
[1.44906559 2.02392394]
[1.09808965 1.28389613]
[1.27828784 2.38870575]
[2.79911065 2.94830836]
[1.33047801 1.53441192]
[2.5538766 1.72098926]
[1.42565413 2.50231096]
[3.18686519 1.71129202]
[2.80344613 1.87373915]]
Generación 25
[[2.01906762 2.00136723]
[2.07934742 2.43250613]
[1.89446298 0.62874045]
[2.19118717 1.28983361]
[1.43938966 2.78994413]
[1.42409891 2.28706104]
[2.72639868 1.54820834]
[0.50852142 1.99835494]
[2.38579999 2.34941743]
[1.67533275 2.63088342]
[1.22920882 0.68371797]
[1.80912008 2.39517979]
[1.70509191 2.5059149 ]
[3.00523491 1.19749159]
[1.82602748 1.76488102]
[2.92322594 1.69983699]
[1.01953492 1.91305785]
[1.34736582 2.59463826]
[2.18748374 2.81280488]
[3.09877138 2.74911112]]
Resultados¶
solution, solution_fitness, solution_idx = ga_instance.best_solution()
print("best_solution: {solution}".format(solution =solution))
best_solution: [2.01906762 2.00136723]
print("best_solution fitness: {}".format(solution_fitness))
print("Valor de f: {}".format(1/solution_fitness))
best_solution fitness: 2736.4004513483387
Valor de f: 0.000365443588312251
solution_idx
0
ga_instance.plot_fitness()
plt.close()
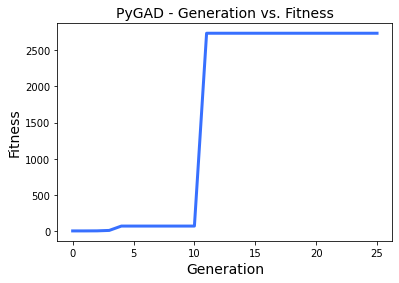
ga_instance.plot_genes(plot_type="scatter")
plt.close()
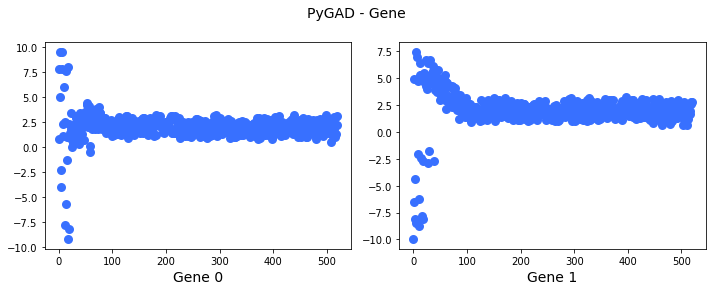
ga_instance.population
array([[2.01906762, 2.00136723],
[2.07934742, 2.43250613],
[1.89446298, 0.62874045],
[2.19118717, 1.28983361],
[1.43938966, 2.78994413],
[1.42409891, 2.28706104],
[2.72639868, 1.54820834],
[0.50852142, 1.99835494],
[2.38579999, 2.34941743],
[1.67533275, 2.63088342],
[1.22920882, 0.68371797],
[1.80912008, 2.39517979],
[1.70509191, 2.5059149 ],
[3.00523491, 1.19749159],
[1.82602748, 1.76488102],
[2.92322594, 1.69983699],
[1.01953492, 1.91305785],
[1.34736582, 2.59463826],
[2.18748374, 2.81280488],
[3.09877138, 2.74911112]])