Algoritmos Genéticos aplicados a la optimización de funciones¶
https://pygad.readthedocs.io/en/latest/
https://pygad.readthedocs.io/en/latest/README_pygad_ReadTheDocs.html
import pandas as pd
import numpy as np
import pygad
Optimizar la función¶
\[f(x,y) = (x-2)^2 + (y-2)^2\]
que tiene un mínimo en \((2,2)\)
def fitness_func(solution, solution_idx):
# Calculating the fitness value of each solution in the current population.
# The fitness function calulates the sum of products between each input and its corresponding weight.
# output = numpy.sum(solution*function_inputs)
# fitness = 1.0 / numpy.abs(output - desired_output)
f_xy = (solution[0]-2)**2 + (solution[1]-2)**2
#print("fitness_func", solution, solution_idx, f_xy)
return f_xy **(-1)
def on_generation(ga):
print("Generación", ga.generations_completed)
print(ga.population)
num_generations = 50
num_parents_mating = 4
fitness_function = fitness_func
sol_per_pop = 20
population_list=[[10,10], [-10,-10], [10,-10], [-10,10]]
num_genes = 2
mutation_num_genes=2
init_range_low = -10
init_range_high = 10
parent_selection_type = "sss"
keep_parents = 1
gene_type=float
crossover_type = "two_points"
mutation_type = "random"
mutation_percent_genes = 10
ga_instance = pygad.GA(num_generations=num_generations,
sol_per_pop=sol_per_pop,
num_parents_mating=num_parents_mating,
fitness_func=fitness_function,
num_genes=num_genes,
mutation_num_genes=mutation_num_genes,
init_range_low=init_range_low,
init_range_high=init_range_high,
parent_selection_type=parent_selection_type,
gene_type=gene_type,
keep_parents=keep_parents,
crossover_type=crossover_type,
mutation_type=mutation_type,
mutation_percent_genes=mutation_percent_genes,
save_solutions=True,
on_generation=on_generation)
ga_instance.run()
Generación 1
[[ 2.5520352 3.47536803]
[-0.71325481 3.50561339]
[-1.1284718 -1.79229247]
[ 3.95183721 -1.20577764]
[ 5.13440685 3.26396596]
[-1.22718245 4.10127828]
[-1.37462706 -0.71091684]
[ 1.03215192 5.65825332]
[ 5.19596239 4.16031416]
[-1.30571292 3.22850443]
[ 0.86142209 3.45317331]
[ 4.79448364 -1.013262 ]
[ 3.51545548 4.96186454]
[-1.32123828 2.55098833]
[-0.27890927 2.74590778]
[ 4.48104688 -2.13947545]
[ 2.93737219 5.59638446]
[ 3.15273001 3.31922808]
[ 0.61761562 2.0160858 ]
[ 3.79090213 -0.38087384]]
Generación 2
[[ 0.61761562 2.0160858 ]
[ 2.45036622 1.13177597]
[ 1.86184174 4.25811034]
[ 2.20750789 3.09624159]
[ 0.91912666 2.36384935]
[ 2.99865567 1.36776367]
[ 1.59610887 2.48547291]
[ 3.18741869 2.50814447]
[ 0.94530622 3.63844755]
[-0.04903989 4.16183809]
[ 2.723214 3.10708606]
[ 0.62033784 3.96636005]
[-0.16731 3.79986999]
[ 2.73668842 1.3435262 ]
[ 2.6724098 2.48257227]
[ 3.86146308 3.63542014]
[ 1.27287571 1.46862933]
[ 0.70934444 2.84295862]
[ 3.87767472 2.99332324]
[ 3.07262297 3.03776578]]
Generación 3
[[1.59610887 2.48547291]
[2.12326085 2.28081713]
[0.53154133 1.80765495]
[0.78897022 1.88913433]
[1.08858389 1.02025875]
[2.33551708 1.59223421]
[0.29977623 2.34130222]
[1.9861752 0.77346838]
[2.32848242 0.70588171]
[2.48952839 2.76086526]
[2.42012769 1.71151346]
[1.38472436 1.92173549]
[1.89488347 1.57768531]
[1.70552342 2.7795935 ]
[0.99671229 2.34168314]
[0.50326094 0.6193038 ]
[2.23533271 0.41781113]
[1.96823268 2.47556607]
[0.56144626 1.75320929]
[3.10058021 0.65133557]]
Generación 4
[[2.12326085 2.28081713]
[1.77271378 1.67547844]
[2.15388026 2.96609426]
[1.44787063 1.11562184]
[1.67621822 1.77106761]
[1.63049972 2.49960533]
[2.83138725 1.68835742]
[3.3527167 3.31076124]
[3.00443134 3.23786788]
[1.55887344 1.63404542]
[1.4911301 1.94228032]
[2.1049362 1.41694133]
[3.07387272 2.5861589 ]
[1.44200579 2.44792167]
[2.52137165 1.49026478]
[1.09559908 1.74156463]
[3.05398079 1.56439127]
[2.1012999 3.18758627]
[1.86030975 3.05873449]
[2.110664 2.64017308]]
Generación 5
[[2.12326085 2.28081713]
[1.70357928 2.55900642]
[2.46186627 1.80580168]
[1.49185402 1.25461256]
[1.4263426 2.56811732]
[2.22953834 1.22154384]
[2.04412897 1.26404788]
[1.65742163 1.60040732]
[1.42652793 1.8783697 ]
[0.7774938 3.254154 ]
[0.91692448 2.4142525 ]
[2.11525082 1.06781783]
[1.57286002 1.82523737]
[1.2261009 1.29798397]
[2.59310095 2.00792707]
[0.93001281 2.67879448]
[2.74166744 1.88625231]
[2.75724045 1.82664595]
[1.8696964 0.83812799]
[0.98037098 1.54256858]]
Generación 6
[[2.12326085 2.28081713]
[2.38763326 1.10540986]
[1.61180728 1.93808752]
[1.65376931 1.11328929]
[0.92988134 2.82733067]
[1.50045209 2.19239788]
[1.41370757 2.62835647]
[1.09665092 0.85021945]
[2.06877068 2.06189909]
[1.42558164 1.42492898]
[2.17005029 2.63152947]
[3.27475463 1.88503361]
[1.78226036 3.26562235]
[2.40314849 1.79100729]
[0.91904221 1.01226642]
[0.85420015 2.57640476]
[2.74450344 0.78444732]
[1.96690233 1.8121199 ]
[2.84375484 2.069528 ]
[3.40940685 1.90267135]]
Generación 7
[[2.06877068 2.06189909]
[3.06671763 2.48617563]
[2.23671195 2.59687734]
[2.02729293 2.45887875]
[2.45884981 1.20065486]
[1.58087774 0.93430902]
[2.2896917 0.97640372]
[1.39517889 1.70455572]
[2.22431854 1.18292365]
[1.68852132 2.0599995 ]
[1.98085777 1.57134383]
[2.24639276 1.47475988]
[1.70673651 2.63167377]
[1.83215293 2.76512071]
[2.21476872 3.08039968]
[1.90365337 1.85816528]
[2.95371336 2.44956508]
[1.36202307 2.56346263]
[1.08790332 3.27111439]
[1.3617 2.79518677]]
Generación 8
[[2.06877068 2.06189909]
[0.92434464 1.1871425 ]
[2.30647048 2.14652438]
[0.94559882 0.67070461]
[2.92377543 0.92952865]
[2.59291899 2.83013909]
[1.4265832 1.65269518]
[1.26597563 1.87598213]
[2.29914089 0.66044881]
[2.57405217 1.40342178]
[0.96926703 2.85382034]
[1.69604061 1.85487991]
[1.21660145 1.11450852]
[1.75595953 2.73905419]
[2.83723674 2.77653441]
[1.07981774 1.84838146]
[1.52427799 2.09161718]
[2.31371342 2.69832905]
[2.20923538 2.64583611]
[1.75396714 1.45422498]]
Generación 9
[[2.06877068 2.06189909]
[1.94119847 2.37805372]
[2.88413979 2.49821998]
[1.35629457 2.56071494]
[1.85534739 2.85806911]
[2.54958901 2.16873943]
[1.1096604 1.9181749 ]
[2.73657395 2.58321299]
[2.19946758 1.45254354]
[2.00919208 2.39637467]
[1.88224461 2.02567632]
[2.89738371 1.49639632]
[2.86796629 2.89799887]
[1.26002935 2.01277941]
[2.32366204 1.54281538]
[1.41289463 1.1654066 ]
[2.18775278 1.58962901]
[1.06844111 2.69001255]
[2.8694687 1.86078711]
[2.74343583 2.18022872]]
Generación 10
[[2.06877068 2.06189909]
[2.90610989 2.44204898]
[2.47666429 3.09189368]
[2.70577581 3.05662132]
[1.73451459 1.50818918]
[1.77209321 2.75460857]
[1.70811226 3.32054356]
[2.45425396 1.9464197 ]
[2.78913663 2.4893121 ]
[1.60737791 1.56344472]
[2.21728362 2.99292188]
[1.67562048 1.64829127]
[1.83998864 3.13304714]
[1.74154085 1.57067089]
[1.22921964 1.94680017]
[2.99189949 1.65029553]
[1.49757525 3.07367063]
[1.74006466 2.40382596]
[2.24842781 2.9754219 ]
[2.53175707 1.65141917]]
Generación 11
[[2.06877068 2.06189909]
[1.6522054 1.69891905]
[2.01452024 1.44366851]
[1.60919069 2.85014868]
[2.30385187 3.35479011]
[2.90672509 2.2097234 ]
[1.51278025 1.17098167]
[2.0758432 1.70041159]
[2.3144854 2.41151314]
[2.24614595 2.75397054]
[2.3014507 2.50646649]
[2.52439172 1.84122563]
[1.1046719 3.05874501]
[2.36500755 2.60687448]
[2.54832192 1.9805081 ]
[0.78536941 1.41826021]
[2.0376036 2.42834135]
[3.19044693 2.28074314]
[1.79156341 2.05455874]
[2.48924383 1.39830782]]
Generación 12
[[2.06877068 2.06189909]
[1.06468535 2.75108772]
[1.7433041 2.07496192]
[2.34641424 1.24029244]
[1.83923123 3.17939408]
[1.30634755 2.81842708]
[1.69030696 2.19028645]
[2.45283918 1.87353016]
[1.63038105 2.32877276]
[1.97585931 1.85358335]
[1.5427521 1.14360208]
[2.68496034 1.65327844]
[2.38115958 2.67702731]
[1.21200791 1.50132685]
[2.1011485 0.83713934]
[2.09483019 1.85476675]
[2.54512778 2.30992765]
[1.84794402 1.96047468]
[2.59178953 1.24822676]
[2.33623657 2.22130098]]
Generación 13
[[2.06877068 2.06189909]
[2.2177366 1.25764046]
[2.94297243 1.77142446]
[2.69323036 1.6466511 ]
[2.34482468 1.91607248]
[1.80272171 2.94031708]
[2.63972465 2.28961912]
[2.18171873 1.93164808]
[2.45351693 2.25330117]
[1.59850825 2.4833091 ]
[2.11527317 2.02267359]
[2.06921727 1.87216194]
[1.37728929 2.5464416 ]
[2.81902135 1.24485626]
[1.02403467 2.67743978]
[1.62152921 1.62769996]
[3.01677142 1.75745319]
[1.09016673 1.16189389]
[1.98511927 1.43294669]
[1.15890535 2.25885317]]
Generación 14
[[2.06877068 2.06189909]
[2.96969724 1.03194965]
[1.30017435 1.88144195]
[1.95440592 2.79175438]
[2.13732498 2.65843542]
[1.74581151 2.48486044]
[1.59909461 1.54900688]
[2.85003981 1.31278919]
[1.5732819 1.88503718]
[1.52549185 1.4086168 ]
[1.16620444 2.83245922]
[1.13676453 2.13853375]
[2.48996596 0.9887214 ]
[1.88883671 2.21349476]
[1.42111854 2.51924591]
[1.94836927 2.70564278]
[2.43777514 1.11434179]
[1.88334111 2.53279756]
[3.00897335 1.58506656]
[2.28785343 2.03954878]]
Generación 15
[[2.06877068 2.06189909]
[2.35875377 2.98971527]
[1.92869855 2.21018354]
[2.75606465 2.31337056]
[2.13838489 1.21050755]
[2.17963179 2.26124412]
[2.59601769 1.99443903]
[2.16105582 1.47175793]
[2.23262964 2.5105003 ]
[2.23677037 1.91880104]
[1.70609184 1.89585579]
[2.00685002 1.27840007]
[0.7474795 1.33253262]
[2.28581803 1.29606978]
[2.31544209 1.85984679]
[2.48611808 3.00900495]
[0.75144662 1.47901576]
[1.14689694 2.86589124]
[1.76732774 2.7968746 ]
[2.08306963 2.26341718]]
Generación 16
[[2.06877068 2.06189909]
[1.97018118 1.49854784]
[3.07073228 2.39055489]
[2.42678169 0.95248874]
[1.65535865 1.64085903]
[2.20193663 1.45766753]
[2.46833244 2.30209416]
[1.90212075 1.13931295]
[2.61923046 2.91942765]
[2.00026979 1.72758542]
[2.7066485 2.47293009]
[1.60645131 2.4088898 ]
[2.3673268 1.50757833]
[2.66677437 3.12435798]
[2.81056026 2.17912491]
[1.32761566 2.40309533]
[3.04528251 1.14887633]
[1.69520073 1.98183684]
[1.9883541 2.85441504]
[2.1617042 2.24026293]]
Generación 17
[[2.06877068 2.06189909]
[2.55982473 2.15589396]
[1.4471433 3.08832827]
[0.77012537 1.75569012]
[1.42615032 2.40067713]
[1.29360871 2.32831087]
[2.65840574 1.9398368 ]
[1.96629665 1.89766709]
[1.44775898 2.18271221]
[1.6355139 2.42859288]
[2.50358691 1.74715934]
[1.95338808 2.6995643 ]
[1.40807357 2.38768731]
[1.5186531 2.63098724]
[2.68537703 2.48060348]
[2.18375655 2.54206225]
[0.88837481 2.04064626]
[2.99756201 1.62642826]
[1.51337337 1.24852984]
[2.08195071 2.45951387]]
Generación 18
[[2.06877068 2.06189909]
[1.08867059 1.5995033 ]
[2.37819796 1.03131079]
[1.43688344 2.82382068]
[2.93273942 1.91703918]
[2.41999274 2.02423554]
[2.55619181 2.33221719]
[2.44148347 2.68106194]
[1.38540445 2.07796696]
[1.25056821 2.3501105 ]
[1.64861876 2.73038813]
[0.69775315 1.73915669]
[1.04801495 2.23706782]
[2.94343816 1.37955014]
[1.36493441 3.40751403]
[2.04941656 1.5253942 ]
[2.67132897 2.40891978]
[2.96265969 2.72068604]
[2.86640864 3.29085654]
[2.64242575 1.68108029]]
Generación 19
[[2.06877068 2.06189909]
[2.1941225 2.2548794 ]
[2.53990045 1.73359602]
[1.82257295 2.23712886]
[2.2652104 1.12440509]
[2.94455215 2.32530549]
[2.88199692 2.50438263]
[1.55746372 1.76765613]
[1.59362792 1.4271333 ]
[1.08675579 1.28412988]
[1.47788644 1.15178334]
[2.08744903 1.81654852]
[2.39495669 1.22591514]
[1.5538705 2.88677754]
[2.45067743 1.4260019 ]
[1.17195066 2.43348978]
[2.17924817 1.3332632 ]
[2.90352338 1.10538309]
[1.5348271 1.42088166]
[1.60374969 2.57277533]]
Generación 20
[[2.06877068 2.06189909]
[1.47950759 2.64209019]
[2.85928403 2.66950746]
[1.03367649 2.66996798]
[1.77000246 2.81241252]
[1.93758903 2.54868629]
[3.06378252 1.70500496]
[2.18287983 2.02951978]
[2.72645507 2.82523981]
[1.5661364 0.91757315]
[2.63181293 1.10348688]
[2.07499821 2.56220068]
[1.72530327 2.53268128]
[2.00818634 1.77420024]
[2.6273953 1.33383568]
[1.30485572 2.82510269]
[2.71482853 2.38806858]
[2.25910084 2.62983288]
[1.74488326 1.541032 ]
[2.10029488 3.12365816]]
Generación 21
[[2.06877068 2.06189909]
[1.23142119 2.06000047]
[1.16757452 1.10527614]
[0.96993335 2.44993784]
[2.11742192 1.18131717]
[1.75821286 1.21187782]
[1.59996427 1.22286367]
[2.73764674 0.92746564]
[2.53622856 0.82391647]
[2.78215951 1.73596961]
[1.43219204 2.72846354]
[0.75846287 1.75974663]
[1.38845336 2.70278404]
[2.45373023 1.17455877]
[2.53999679 1.24251815]
[1.27543882 2.53486374]
[1.80448067 2.06478776]
[2.28655586 1.68275094]
[1.22127068 2.67848274]
[1.76240681 1.41501612]]
Generación 22
[[2.06877068 2.06189909]
[1.74149103 1.26757707]
[0.9153644 2.5874945 ]
[2.52646641 2.20707002]
[2.59947555 0.83424854]
[1.20105605 1.71747352]
[3.0403406 2.37183498]
[2.34515837 0.9935297 ]
[2.34030505 2.3314224 ]
[2.36150882 2.52796668]
[1.44804472 1.09516946]
[1.62064706 0.92806021]
[0.95828666 2.70335783]
[2.58868673 2.50110052]
[2.23252476 2.10667767]
[1.57764768 1.39563085]
[1.13164549 0.54189534]
[2.04113965 1.79265289]
[1.63470519 1.25920384]
[1.77072053 1.82059773]]
Generación 23
[[2.06877068 2.06189909]
[1.62601785 1.96430547]
[2.54182436 1.5466068 ]
[1.39000858 1.58869695]
[1.73380381 2.86608943]
[2.41974026 1.06384524]
[2.90163347 2.29625052]
[1.70815794 1.85394364]
[1.86884396 2.29246488]
[1.88941003 2.45650422]
[2.75090742 1.7232625 ]
[1.8244364 1.66217821]
[0.82703803 2.71746619]
[1.30815539 2.65488171]
[1.23040889 1.37625441]
[2.29480006 2.54695325]
[1.45752553 1.84523627]
[2.41570302 2.0200836 ]
[1.70219201 2.07876753]
[1.64654711 2.96949648]]
Generación 24
[[2.06877068 2.06189909]
[1.58033381 2.77254196]
[1.77909879 2.77248436]
[1.2474502 2.70742624]
[1.64531232 2.80660315]
[1.10084301 1.6501029 ]
[1.15355584 1.30204333]
[2.56893429 1.80145673]
[2.16126912 2.74795518]
[1.61878448 3.05267491]
[1.13367021 2.76740164]
[2.22336393 1.52391369]
[1.33033997 1.55944678]
[1.45356008 1.83288151]
[2.47076723 2.12454455]
[2.72735593 1.7396164 ]
[0.94304272 1.27383387]
[1.53338707 1.69981807]
[1.67032223 1.31617681]
[1.94304001 2.43460106]]
Generación 25
[[2.06877068 2.06189909]
[2.15607843 2.69073435]
[2.90242053 2.96271263]
[1.76111973 3.09836505]
[2.35594225 2.19440775]
[2.24362875 2.94752776]
[2.62122022 2.95634245]
[1.6198141 2.88910222]
[1.76059912 0.74828173]
[2.58070295 2.69740564]
[2.80025468 3.31071866]
[3.41397065 0.59486953]
[2.97561595 2.267892 ]
[2.40710126 1.98270605]
[2.37956017 2.87300955]
[2.72681404 2.43994682]
[2.38285416 0.93796132]
[2.89893371 2.12894605]
[2.34727897 1.98019921]
[3.05692375 0.92831905]]
Generación 26
[[2.06877068 2.06189909]
[1.42895851 1.05939495]
[2.97290645 2.14670852]
[1.53532583 1.91128667]
[1.07071411 1.26038986]
[3.01489104 1.86525058]
[2.69306667 2.97120859]
[1.64334845 1.89454012]
[2.93580838 2.65040892]
[2.26183985 1.92524973]
[1.38882818 2.87883207]
[2.69921317 1.75126603]
[1.90393042 1.85696965]
[3.0805367 1.87968246]
[1.53820818 2.0578507 ]
[2.96670548 3.00017398]
[3.08973716 2.327303 ]
[1.44783201 1.92758498]
[1.67903121 2.05074187]
[2.69182243 1.98916349]]
Generación 27
[[2.06877068 2.06189909]
[2.39087147 2.2235019 ]
[1.43968597 2.28024783]
[2.29729721 1.26462755]
[1.88507176 2.8251631 ]
[1.97746821 1.47582914]
[2.81295438 1.739149 ]
[1.68732044 2.97898853]
[2.18353192 2.00507057]
[1.28751983 2.00041447]
[1.42213901 1.2892188 ]
[1.91057686 1.10486537]
[1.17258588 1.71807176]
[1.14724282 1.63878509]
[2.48130828 1.64785472]
[3.10989969 2.99109943]
[1.5243988 3.0313616 ]
[1.271198 1.65967011]
[1.52520746 2.88122549]
[2.26826243 1.09934052]]
Generación 28
[[2.06877068 2.06189909]
[1.95040653 2.89174185]
[3.06149811 1.90813632]
[2.43769907 2.07838375]
[1.66669544 1.93415579]
[1.07063294 1.4232031 ]
[1.4157222 2.3640606 ]
[2.22171755 2.24253284]
[1.55558412 1.09751461]
[1.40084208 1.70845079]
[3.13632989 2.74956423]
[3.33649147 2.37726852]
[2.27473225 0.79887637]
[1.80487982 2.23847726]
[1.48129624 2.34430427]
[2.7271298 2.17023795]
[1.13007412 1.83831376]
[1.80781729 1.94741439]
[3.31220329 1.52379706]
[2.09972601 1.51460126]]
Generación 29
[[2.06877068 2.06189909]
[2.03804066 2.37290942]
[1.18352218 3.10343058]
[1.52182875 3.06955723]
[2.17308857 3.15209752]
[1.1078023 2.01645302]
[1.78794226 1.46994443]
[1.97070806 2.53236498]
[2.14653193 1.53763022]
[0.93536906 2.77998658]
[2.78653618 2.76975196]
[2.53813556 1.94317591]
[1.82442743 1.99315896]
[1.40553594 1.52595021]
[1.64177573 2.00604143]
[1.80633606 1.47543123]
[1.55139462 1.61551305]
[2.48724748 2.07497733]
[1.67360747 2.1648565 ]
[0.86505433 2.70241605]]
Generación 30
[[2.06877068 2.06189909]
[1.32355915 2.47543789]
[2.61638966 1.33307955]
[0.70080107 1.33089181]
[2.08720364 2.8540799 ]
[2.01881459 2.81539437]
[2.28125088 1.62375111]
[1.21755107 2.47854922]
[2.9010724 2.20005447]
[1.58618633 2.08245635]
[2.38491309 1.77900471]
[0.71227492 1.10232655]
[1.17704769 1.10689406]
[2.32170763 1.75268171]
[2.38080247 2.34646873]
[1.19138929 2.90280596]
[1.70536919 2.84243143]
[1.76874852 1.60352194]
[2.75225622 2.12026835]
[2.33459534 1.98593484]]
Generación 31
[[2.06877068 2.06189909]
[1.38338176 2.62180682]
[1.35250246 1.57764559]
[1.48487718 2.3834453 ]
[1.19302841 2.66467272]
[3.33228109 2.2767247 ]
[2.27921626 1.17871499]
[2.27435092 0.99866499]
[2.33163594 2.03728793]
[2.29014669 1.9648942 ]
[2.03296789 1.26683806]
[0.78012194 0.76772648]
[1.63119713 1.37059473]
[1.32370653 2.55514177]
[2.51448412 1.64127822]
[2.19687743 1.55058863]
[1.75219284 1.27922939]
[2.67757242 1.72428946]
[1.82526645 2.72418714]
[2.05157644 1.96849256]]
Generación 32
[[2.05157644 1.96849256]
[2.3839435 1.4564658 ]
[1.32109786 1.89374291]
[2.20539472 1.30573659]
[2.30476252 2.60221706]
[2.23831287 2.04657387]
[3.02269049 1.84018419]
[2.33456264 2.19246368]
[2.59550706 2.58260821]
[1.57101192 2.13189081]
[2.38757332 1.78440689]
[1.68069468 1.62561578]
[2.32557391 1.25829626]
[1.15543215 0.97830997]
[2.63922481 1.81019983]
[2.79201838 2.03040837]
[2.13574612 2.80402536]
[1.21710723 2.88468877]
[1.32798237 1.032894 ]
[3.15412706 2.78021289]]
Generación 33
[[2.05157644 1.96849256]
[1.32973324 2.64887693]
[3.04087725 1.60940761]
[2.44696159 3.08043811]
[2.86369238 2.6452143 ]
[1.06932449 2.20253098]
[2.67640069 1.8241978 ]
[2.89106956 2.69575713]
[1.38836001 1.44451391]
[2.73815868 1.20281649]
[2.22731769 2.55322417]
[2.73494309 3.1298685 ]
[3.0538595 2.33889404]
[2.27620574 2.69549523]
[1.62261322 2.11512151]
[2.85853576 2.52015784]
[1.57237129 1.27706053]
[2.91766389 1.77537818]
[1.43420797 2.81963548]
[2.05567232 1.30953617]]
Generación 34
[[2.05157644 1.96849256]
[1.93122555 2.89202369]
[2.4924658 2.65201006]
[2.83058117 3.14029598]
[2.20509737 1.33647007]
[1.12125571 2.86699933]
[2.24997919 1.21288283]
[1.64146447 0.39270062]
[1.21741289 1.65245068]
[1.52001507 1.29392029]
[1.7691154 3.07246044]
[1.92943606 2.83715125]
[1.29561401 0.45650184]
[2.93111079 1.84801982]
[1.88594839 3.0287656 ]
[1.61452728 1.08255316]
[1.33334489 0.72372015]
[1.963523 2.15164783]
[2.23827025 3.05184529]
[2.99298654 3.1526457 ]]
Generación 35
[[2.05157644 1.96849256]
[1.29139061 1.2117064 ]
[1.95919779 1.73406424]
[2.44329747 1.56032397]
[2.11188537 2.4552857 ]
[1.24803069 2.57538068]
[2.17154729 1.76530959]
[3.29003019 1.82987591]
[2.69421736 1.6059136 ]
[0.97997068 1.74832927]
[2.96757154 2.81483218]
[3.2342879 1.84761565]
[3.45788698 1.13276444]
[2.3936588 2.33308626]
[1.89687951 0.83091306]
[3.03053618 1.87639829]
[2.23131342 2.4487165 ]
[1.35782294 1.34568908]
[1.70164828 1.60764303]
[2.66744982 2.27137538]]
Generación 36
[[2.05157644 1.96849256]
[2.11642688 1.39023153]
[1.68449825 0.86397156]
[1.80287366 3.29901891]
[1.29260193 1.4134245 ]
[2.15853117 1.42193958]
[2.43029843 1.64239719]
[3.02732379 2.48203797]
[3.01817797 2.47704499]
[2.52146161 2.52270288]
[2.18408394 1.04967129]
[1.24841309 2.04276079]
[1.8517579 2.72795354]
[2.21438825 1.17028339]
[2.61296938 1.25889215]
[2.45467759 3.18333204]
[1.10589673 2.21811478]
[1.57026949 2.54919108]
[2.29288959 1.6614814 ]
[2.67930953 2.08644109]]
Generación 37
[[2.05157644 1.96849256]
[1.4227642 1.0846678 ]
[3.18294632 2.05089775]
[1.31621042 1.15821277]
[2.15848369 1.01664181]
[1.90371908 1.89199732]
[1.4568125 0.78915598]
[1.36414605 1.66301094]
[1.94617926 1.38214036]
[3.28591157 1.74840137]
[1.81065651 1.27757761]
[2.02472847 1.42573435]
[2.75512707 2.92161735]
[1.16706808 1.97400181]
[1.97414282 1.21737572]
[1.55266728 1.93980673]
[2.50359032 2.22152815]
[1.16156499 1.34810355]
[2.68081693 2.41062495]
[1.39717869 1.52332847]]
Generación 38
[[2.05157644 1.96849256]
[2.69436813 2.55763763]
[1.78755969 1.01711741]
[2.72544224 1.78901309]
[3.03346713 2.33499813]
[2.24826245 1.57406933]
[2.17474205 1.26189732]
[1.35590447 2.35082435]
[1.81220081 2.51157426]
[1.10550284 1.48666653]
[2.62138607 1.05270144]
[1.90020454 2.52230473]
[1.24893022 2.45087433]
[1.28895924 1.7687937 ]
[1.5083992 2.08205216]
[2.29301722 2.23549575]
[2.79231068 2.90731407]
[1.67634187 1.817469 ]
[0.67651942 1.50953377]
[2.06596306 1.50497839]]
Generación 39
[[2.05157644 1.96849256]
[0.70565129 2.79201235]
[1.93028923 2.51963948]
[3.00167714 2.24942357]
[1.86227137 2.27804012]
[3.04012052 0.96861859]
[2.17117031 1.54595803]
[2.85824237 1.68558421]
[2.3980553 0.93029373]
[1.26907805 2.43562174]
[1.98454257 2.88413287]
[1.92900112 0.94036678]
[1.85246828 2.37894198]
[1.30231761 1.90632469]
[2.69916115 2.04195266]
[1.46203278 2.9171605 ]
[2.37774597 2.51790198]
[0.97537597 1.94661729]
[1.92656617 2.77704293]
[1.37550373 1.1258132 ]]
Generación 40
[[2.05157644 1.96849256]
[1.33870412 2.78157493]
[2.23863616 3.12621087]
[2.4430537 2.06538673]
[1.6569633 2.84285563]
[1.09445551 2.70332637]
[0.98373516 2.60452247]
[1.95187691 3.23942377]
[3.0844227 1.28999657]
[2.47419496 2.30191342]
[2.52894264 3.08048683]
[2.00987226 0.7653511 ]
[1.89311174 1.09362199]
[3.01695609 2.87520054]
[1.90505417 3.06810452]
[2.07933433 1.58461586]
[1.55683533 1.49292335]
[1.7203661 1.81056766]
[1.08339889 1.64284759]
[1.30291873 2.02857798]]
Generación 41
[[2.05157644 1.96849256]
[1.77516336 2.66558765]
[1.60836152 2.18795464]
[1.18898733 2.35264644]
[2.72595114 1.54683395]
[1.12762857 1.2714635 ]
[2.3971072 2.19321998]
[1.62824444 1.98903286]
[2.75082628 1.41087332]
[2.29817268 1.17212187]
[2.07647208 0.81170205]
[2.47288001 0.84283341]
[1.89543615 2.29961881]
[2.06250778 0.89742976]
[1.12373102 1.91417318]
[2.77392226 2.4424642 ]
[2.50239725 1.79162662]
[1.61395769 1.90249238]
[2.19495727 0.70376995]
[2.26627041 1.30509829]]
Generación 42
[[2.05157644 1.96849256]
[2.63685672 1.25248324]
[0.93972866 1.7858919 ]
[1.0032755 2.27776743]
[2.56809416 1.18993849]
[2.45696862 1.34776839]
[2.5682721 1.36964428]
[0.74375842 2.49898728]
[1.39353067 0.97982809]
[1.57558136 3.23119881]
[1.95376632 2.43402011]
[1.75326243 2.68462134]
[2.93572014 1.48897239]
[1.57426609 1.4211292 ]
[1.2644728 1.59139834]
[2.44409122 2.54240635]
[1.15488211 1.11088544]
[1.49501302 2.64795394]
[1.7899127 2.54943002]
[1.88788943 1.11703616]]
Generación 43
[[2.05157644 1.96849256]
[0.97654778 2.3743594 ]
[1.66012887 2.77021184]
[2.59017531 3.27212751]
[1.85949734 2.01684668]
[1.35053073 1.93716619]
[2.63584964 1.83198133]
[2.6792221 2.45598465]
[2.82171415 2.44319383]
[1.84912882 1.23633602]
[0.87264097 1.49897788]
[3.22906129 1.62623492]
[2.30532888 2.17403451]
[1.79567183 1.9137139 ]
[1.05712756 1.6191492 ]
[1.25779137 3.105534 ]
[2.14416627 3.49228586]
[1.28913602 2.57640877]
[1.85331201 3.51925007]
[1.14279046 3.08919366]]
Generación 44
[[2.05157644 1.96849256]
[1.8639897 1.73998743]
[1.97859188 1.74181441]
[1.76759794 1.79303506]
[2.85092484 2.99027365]
[1.27692974 2.18260335]
[2.24935691 2.23932185]
[2.34837632 1.99980267]
[1.8925676 2.22097562]
[2.05739274 2.84225309]
[2.35776189 2.80543821]
[1.21128103 2.20532981]
[1.48592583 2.79979204]
[2.75728945 1.38393715]
[1.83068708 1.28643419]
[1.76419942 2.00699037]
[1.46544162 1.09547059]
[1.65718849 1.67478212]
[2.06492052 1.21566928]
[0.8446988 2.44443552]]
Generación 45
[[2.05157644 1.96849256]
[1.97482713 2.08253804]
[1.45979749 1.93785915]
[1.52472782 1.67382967]
[2.93153569 2.51415557]
[1.41400728 2.30684808]
[2.70760294 1.761331 ]
[2.76271638 2.28740654]
[2.05456932 2.56786802]
[1.32394984 1.53732538]
[2.24864023 1.10734452]
[2.21939325 2.91368902]
[2.86384451 2.37644504]
[1.68184517 2.46372363]
[2.89126972 2.90765964]
[1.97345002 2.41974493]
[1.41510607 1.46131588]
[2.90130136 2.94343652]
[1.60353752 1.56814248]
[1.98308382 3.17992032]]
Generación 46
[[2.05157644 1.96849256]
[1.16171382 1.16300248]
[2.53546627 1.59581251]
[1.09860757 2.41334225]
[1.41574364 1.11016551]
[2.91140469 2.62843369]
[1.90720535 3.30561003]
[1.76480638 2.13891389]
[2.24989761 1.11125446]
[1.45021073 2.72496611]
[2.46041649 1.67967383]
[1.56155353 3.09599271]
[2.09077645 2.73938272]
[1.21372665 1.54250919]
[1.29122471 2.29129384]
[1.67028582 1.77464969]
[0.5676241 1.09388521]
[1.99011955 1.14835569]
[1.19996651 2.6414499 ]
[0.97489653 1.45613201]]
Generación 47
[[2.05157644 1.96849256]
[1.06573591 2.96324906]
[1.01943106 1.41796645]
[2.24887219 1.36517915]
[3.44722634 1.82754778]
[2.29010299 2.18507695]
[1.87055447 2.50097112]
[3.28128619 2.51511985]
[2.22755733 1.99157891]
[1.84975457 1.77640349]
[1.26069162 1.62925247]
[3.35840584 2.23562883]
[1.7834064 1.09672839]
[1.17527576 1.73921602]
[1.93215772 2.14968247]
[2.32955323 1.20239242]
[3.15677164 1.05214508]
[2.54119831 2.89844099]
[1.92586457 1.78675529]
[1.58016011 0.72762864]]
Generación 48
[[2.05157644 1.96849256]
[2.29594807 2.34088782]
[1.33506359 2.7167955 ]
[1.12447623 1.17339595]
[3.0083045 1.58452695]
[1.8943948 1.32263914]
[1.11237587 1.89093402]
[1.33828455 2.08738461]
[2.01298176 2.1246781 ]
[1.78830982 1.56138085]
[1.67085698 2.62026383]
[2.04848226 2.07114777]
[1.70011211 1.47556877]
[2.58973146 1.70457772]
[2.33743571 1.07518273]
[2.58915116 2.18590387]
[1.5854801 1.74127748]
[1.49157615 2.6139259 ]
[2.41495014 1.21416949]
[2.0376188 2.31699857]]
Generación 49
[[2.05157644 1.96849256]
[2.76124992 2.39559687]
[2.19530243 2.35048078]
[2.16800488 1.98310385]
[1.60647168 1.83434557]
[2.75497481 1.2062074 ]
[1.45006966 2.67598655]
[1.08556437 1.99276484]
[1.06734175 2.8282833 ]
[1.96334192 2.62571713]
[2.21699691 2.58380297]
[2.6985598 1.90823487]
[2.0961333 1.57947851]
[2.09301094 2.29572038]
[2.91119079 2.95666892]
[2.60031083 2.72851772]
[1.86312573 3.17239807]
[1.34478122 2.81698348]
[2.2975798 2.83554308]
[1.16986039 2.17418266]]
Generación 50
[[2.05157644 1.96849256]
[2.84497482 1.57075569]
[1.32631589 2.55239625]
[1.62424411 2.40296144]
[2.4328835 1.99324261]
[1.22828911 1.07032933]
[2.26985768 2.053073 ]
[2.10458936 2.69929323]
[2.55913393 2.73335593]
[1.62490293 2.79328237]
[2.2364156 1.61251887]
[1.81435189 2.66448855]
[2.59301755 2.86333395]
[1.24363515 1.18594392]
[2.36674353 2.63412592]
[1.30081512 1.37776782]
[2.11992177 1.60572467]
[2.23671618 2.09420383]
[2.46613593 1.76692209]
[2.49944213 2.9553448 ]]
/home/carlos/.local/lib/python3.8/site-packages/pygad/pygad.py:795: UserWarning: Use the 'save_solutions' parameter with caution as it may cause memory overflow when either the number of generations, number of genes, or number of solutions in population is large.
if not self.suppress_warnings: warnings.warn("Use the 'save_solutions' parameter with caution as it may cause memory overflow when either the number of generations, number of genes, or number of solutions in population is large.")
solution, solution_fitness, solution_idx = ga_instance.best_solution()
print("best_solution: {solution}".format(solution =solution))
best_solution: [2.05157644 1.96849256]
ga_instance.plot_fitness()

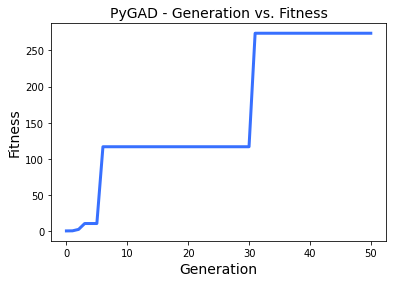
ga_instance.plot_genes()


import random
import matplotlib.pyplot as plt
%matplotlib inline
class AlgoritmoGenetico(object):
"""Algoritmo Genético.
Parametros
------------
num_generations=Nº de generaciones a producir
sol_per_pop=Nº de individuos por generación
num_parents_mating=Nº de padres que se cruzan
fitness_func=Calcula la fortaleza de cada individuo
num_genes=Nº de genes por individuo
mutation_num_genes=Nº de genes que mutan
init_range_low=Rango de Inicio desde
init_range_high=Rango de Inicio hasta
parent_selection_type=Tipo de selección del padre
gene_type=Tipo de gen (int, float)
keep_parents=Padres que se mantienen
crossover_type=Tipo de cruce
mutation_type=Tipo de mutación
mutation_percent_genes=Porcentaje de genes que mutan
on_generation=Función a llamar al final de cada generación
Atributos
-----------
"""
def __init__(self, num_generations, sol_per_pop, num_parents_mating, fitness_func, num_genes,
mutation_num_genes, init_range_low, init_range_high, parent_selection_type, gene_type,
keep_parents, crossover_type, mutation_type, mutation_percent_genes, on_generation):
self.num_generations = num_generations
self.sol_per_pop = sol_per_pop
self.num_parents_mating=num_parents_mating
self.fitness_func = fitness_func
self.num_genes = num_genes
self.mutation_num_genes = mutation_num_genes
self.init_range_low = init_range_low
self.init_range_high = init_range_high
self.parent_selection_type = parent_selection_type
self.gene_type = gene_type
self.keep_parents = keep_parents
self.crossover_type = crossover_type
self.mutation_type = mutation_type
self.mutation_percent_genes = mutation_percent_genes
self.on_generation = on_generation
self.Num_generacion = 0
self.poblacion = [ [ random.randint(self.init_range_low, self.init_range_high) for i in range(self.num_genes)] for j in range(self.sol_per_pop)]
self.fortaleza = [ self.fitness_func(individuo) for individuo in self.poblacion]
def run(self):
self.optimos= []
for t in range(self.num_generations):
new_poblacion = []
for k in range(self.sol_per_pop): ## Se crea una nueva poblacion
padre1, padre2 = self.SeleccionCompetitiva(self.poblacion, self.fortaleza)
hijo = self.Cruzar(padre1, padre2)
hijo = self.Mutar(hijo)
new_poblacion.append(hijo)
self.fortaleza, self.poblacion = self.poblacionResultante(self.poblacion, self.fortaleza, new_poblacion)
self.Num_generacion = t+1
self.optimos.append(self.MejorSolucion())
if t%10==0: self.on_generation(self)
return
def poblacionResultante(self, poblacion1, fortaleza1, poblacion2):
fortPoblacion = []
for fort, indiv in zip(fortaleza1, poblacion1):
elem = [fort]
for gen in indiv:
elem.append(gen)
fortPoblacion.append(elem)
for indiv in poblacion2:
elem = [self.fitness_func(indiv)]
for gen in indiv:
elem.append(gen)
fortPoblacion.append(elem)
fortPoblacion.sort() ## Se ordena por fortaleza
fortPoblacion = fortPoblacion[-self.sol_per_pop:] ## Se toma la población de más fortaleza
new_fortaleza = []
new_poblacion = []
for elem in fortPoblacion:
new_fortaleza.append(elem[0])
indiv = []
for i in range(1, len(elem)):
indiv.append(elem[i])
new_poblacion.append(indiv)
return new_fortaleza, new_poblacion
def Mutar(self, individuo):
prob_mutar = random.randint(0, 100)
if prob_mutar > self.mutation_percent_genes:
return individuo ## Si no cumple la probabilidad de mutar no se hace nada
pos_mutar = random.randint(0, self.num_genes-1)
individuo[pos_mutar] = random.uniform(self.init_range_low, self.init_range_high)
return individuo
def Cruzar(self, padre1, padre2):
#Hacemos una combinación convexa aleatoria entre los 2 puntos de cada padre
k = random.randint(1,10)
hijo = [ padre1[i] + (k/10)*(padre2[i]-padre1[i]) for i in range(self.num_genes)]
return hijo
def SeleccionCompetitiva(self, poblacion, fortaleza):
# Se seleccionan aleatoriamente 2 parejas de individuos de la población
iInd1 = self.SeleccionRandomUnica(0, len(poblacion)-1, [])
iInd2 = self.SeleccionRandomUnica(0, len(poblacion)-1, [iInd1])
iInd3 = self.SeleccionRandomUnica(0, len(poblacion)-1, [iInd1, iInd2])
iInd4 = self.SeleccionRandomUnica(0, len(poblacion)-1, [iInd1, iInd2, iInd3])
iPar1 = iInd1 if fortaleza[iInd1]>fortaleza[iInd2] else iInd2
iPar2 = iInd3 if fortaleza[iInd3]>fortaleza[iInd4] else iInd4
# De cada pareja se ha selecciona el de más fortaleza
return poblacion[iPar1], poblacion[iPar2]
def SeleccionRandomUnica(self, ini, fin, lstAnt):
seguir=True
c=0
while (seguir):
ix = random.randint(ini, fin)
c+=1
try:
if lstAnt.index(ix) < 0 or c==100:
seguir=False
except Exception as e:
seguir=False
return ix
def MejorSolucion(self):
ix = self.fortaleza.index(max(self.fortaleza))
return self.poblacion[ix]
def PlotOptimos(self):
plt.figure(figsize=(10, 7))
x = [optimo[0] for optimo in self.optimos]
y = [optimo[1] for optimo in self.optimos]
plt.plot(x, y)
plt.plot(x[-1], y[-1], '*', color='red')
plt.show()
def this_fitness(solution):
f_xy = (solution[0]-2)**2 + (solution[1]-2)**2
#print("fitness_func", solution, f_xy)
return f_xy **(-1)
def on_generation(ga):
print("Generación", ga.Num_generacion)
poblac_format = [ [ float('{:.2f}'.format(gene)) for gene in individuo] for individuo in ga.poblacion]
print("Población", poblac_format)
population_list=[[10,10], [-10,-10], [10,-10], [-10,10]]
myGA = AlgoritmoGenetico(num_generations=100,
sol_per_pop=40,
num_parents_mating=4,
fitness_func=this_fitness,
num_genes=2,
mutation_num_genes=2,
init_range_low=-10,
init_range_high=10,
parent_selection_type="sss",
gene_type=float,
keep_parents=1,
crossover_type="two_points",
mutation_type="random",
mutation_percent_genes=15,
on_generation=on_generation)
myGA.run()
Generación 1
Población [[-0.4, -5.4], [9.0, 5.0], [-4.8, 5.2], [-1.8, -4.4], [-4.2, -1.2], [-4.5, 4.02], [3.4, -4.6], [4.8, -4.0], [-3.0, 6.0], [-2.0, -3.0], [-3.0, -1.0], [-3.0, -1.0], [6.0, -2.2], [0.4, -3.4], [-3.0, 4.0], [-3.2, 3.1], [-0.46, -2.7], [7.0, 3.0], [-2.2, 4.8], [-2.0, 5.0], [-2.6, 3.8], [0.7, -2.4], [-2.5, 1.5], [5.0, 5.0], [5.0, 5.0], [-2.2, 1.97], [4.9, 4.9], [2.0, -1.5], [4.0, -0.5], [-1.0, 3.0], [5.0, 2.2], [2.2, 5.0], [0.6, -0.6], [4.0, 0.0], [4.0, 4.0], [2.4, -0.4], [0.3, 0.5], [4.1, 2.8], [1.0, 3.0], [1.4, 2.0]]
Generación 11
Población [[1.98, 2.01], [1.98, 2.02], [1.98, 1.99], [1.98, 2.02], [1.97, 2.0], [1.97, 2.0], [1.97, 2.0], [1.99, 2.02], [1.99, 2.02], [1.98, 1.99], [1.98, 2.01], [1.98, 2.01], [1.98, 2.01], [1.98, 2.02], [1.98, 2.01], [1.99, 2.02], [1.98, 2.01], [1.99, 2.02], [1.98, 2.0], [1.98, 2.01], [1.99, 2.01], [1.98, 2.0], [1.99, 2.02], [1.98, 2.0], [1.99, 2.02], [1.98, 2.01], [1.99, 2.01], [1.99, 2.01], [1.98, 2.0], [1.99, 2.02], [1.98, 2.0], [2.0, 2.02], [1.99, 2.01], [1.98, 2.0], [1.98, 2.0], [2.0, 2.02], [2.0, 2.02], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01]]
Generación 21
Población [[2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01]]
Generación 31
Población [[2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01]]
Generación 41
Población [[2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01]]
Generación 51
Población [[2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01]]
Generación 61
Población [[2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01]]
Generación 71
Población [[2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01]]
Generación 81
Población [[2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01]]
Generación 91
Población [[2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01], [2.0, 2.01]]
mejor= myGA.MejorSolucion()
print("Mejor Solucion", mejor)
Mejor Solucion [1.9961868155201496, 2.009474853595225]
myGA.PlotOptimos()
